How To Defer Parsing Of JavaScript In WordPress (4 Methods)
Deferring JavaScript parsing is a quick and simple way to optimise your WordPress site’s speed and can make a massive difference to your SEO and User Experience.
What does it mean to defer parsing of JavaScript?
Ok, let’s start by defining some of the key terms we’ll be talking about in this post.
Parsing means to analyse a text and arrange it into logical components. In the case of a website, this means your browser reads the lines of code that make up your site and arranges them into the elements that appear on the screen.
JavaScript is a programming language that can be used to add interactive elements to websites. Basically, anything on a page that isn’t static is probably using JavaScript.
JavaScript files are bigger than other elements (like HTML) so they take longer to parse. While they are being downloaded and executed, the rest of the site rendering is paused. This is known as render-blocking. Render-blocking causes slower loading times which can be enough to make visitors click away from your site.
Furthermore, slow load times can have a negative impact on your SEO. As Google continues to update its ranking system, page speed is now factored into your rank, with slower sites being ranked lower.
To find out if your site is suffering from render-blocking resources, you can use a variety of performance-testing tools like Google’s PageSpeed Insights or GTMetrix. These tools will identify elements that are hindering your site’s performance and tell you if deferring JavaScript will help.
Defer vs Async
Before we look at the different ways to defer JavaScript parsing, it’s important to understand the difference between defer and async. These are two commands that are used to get around render-blocking but with slight differences between them.
When a page is being parsed and encounters a render-blocking script, async and defer both allow the browser to download the script while still parsing the rest of the page. However, for both commands, the render will still stop to wait for the script to be executed.
The difference between async and defer is in the way they execute the scripts.
When loading a script without attributes, the HTML will be parsed until it reaches the script file. At this point, parsing will stop and the script is downloaded and executed. Once this is done, the parsing resumes.

Async: The script is loaded parallel to the HTML and then parsing is paused as soon as the script is ready to be executed.

Defer: Downloads script alongside the rest of the HTML and waits for everything else to be parsed before executing.

Generally speaking, defer is used more often. The reason for this is that scripts will often be dependent on other elements on the page or on a fully parsed DOM (Document Object Model). In this case, the rest of the HTML will need to be loaded for the script to work correctly.
Async is used in scenarios where your script is independent of other elements and/or it doesn’t matter when it is executed.
When to Use Defer over Async
Even though in many cases, using either defer or async would make no significant difference, it is important to know where defer works better than async
The core principle of a deferred script is that it waits for the entire DOM to be parsed first before it is executed. It does not interrupt or block rendering; this quirk does come with its own sets of drawbacks too.
Defer is best used when your script requires the complete DOM parsed, using Async in such scenarios might cause the “Element not found” error as sometimes a script can be executed without the required DOM elements.
So whenever you have a script with javascript that will only function after the DOM has been loaded, it’s best you use defer over Async.
Another reason why you might want to use defer over async is that it respects the sequence in which scripts are placed.
In the above example, all the scripts will be executed from top to bottom one at a time.
Async on the other hand does not respect the sequence you place your scripts and will execute scripts unpredictably.
Thus, when you have a script that requires another first being executed to work, using defer grants you control over the order of execution.
When to Use Async Over Defer
Async works best in scenarios where a script doesn’t need the DOM or depends on any other existing scripts to function.
A good example would be embed scripts from external services like MailChimp and Google analytics; since most embeds don’t require existing scripts or DOM elements from your website, using the async attribute on them makes a lot of sense
3 Methods to defer parsing of JavaScript in WordPress
There are 3 main ways to defer JavaScript parsing.
The first method is to simply move the offending script lower down in the code. HTML is parsed top to bottom so if the script is placed near the end it won’t be read until everything else has been parsed.
The other methods involve adding either defer or async attributes in the code to tell the browser how to parse the scripts. And then there’s “The Varvy Method” but we’ll get to that in a bit.
Defer parsing of JavaScript with WordPress plugins
WordPress users will know that one of the blessings of the platform is the multitude of plugins at your disposal that make site customisation easy and accessible. So, if you don’t fancy manually altering any code, these plugins have got you covered.
This plugin is lightweight, simple, and best of all: free. Once installed, you can choose which scripts to apply defer or async to.
This site acceleration plugin is designed to make your site run as fast as possible, so obviously it includes the option to defer JavaScript. Simply go to “Page Optimisation” then “JS Settings”, turn on the “Load JS Deferred” option and you’re good to go!
In the WP Rocket menu, navigate to the File Optimisation tab, check the box marked “Load JS Deferred” and let the plugin work its magic.
Manually defer parsing
Alternatively, you can manually edit the functions.php file of your WordPress site to assign the defer attribute.
Be careful when making changes to site files. Errors in the code could end up locking you out of your site.
Before editing the functions.php file, download a backup copy of the file so you can restore it if anything goes wrong. Or, for extra security, make a complete backup of your site.
How to access functions.php
WordPress Theme Editor
WordPress comes with a built-in text editor for making changes to your theme files. To make changes to functions.php in the theme editor do the following:
- Log in as admin and access your WordPress dashboard
- Go to Appearance>Theme Editor
- Select the theme you want to edit from the drop-down menu
- Under Theme Files click on the functions.php file
FTP Client
If you can’t access the functions file through the theme editor, you can find it in your FTP client.
- Open your FTP client and locate the public_html folder
- From here, go to wp-content and open the themes folder, then open the folder of the theme you want to edit
- Inside the theme folder, locate the functions.php file
- Download a backup copy of the functions.php file to your computer before editing it
- Open the original functions.php in a code editor such as Notepad++
Edit functions.php to defer parsing
Once you’re in the functions.php file, go to the bottom of the file by pressing CTRL+END.
Then, add the following code snippet into the file:
The Varvy Method: The Ultimate Defer Script
If you’re looking for a very simple approach to getting rid of render-blocking scripts via the defer method, the Varvy method created by Patrick Sexton does just that.
Instead of complicating things, Patrick’s method works by aggregating all your scripts into a single external file which is only executed when the page is completely loaded.
So, instead of relying on individual defer attributes throughout your HTML, you just drop in one script that points to the external file with a defer attribute present.
“Defer.js” in the above code snippet would be the file housing all the scripts you want deferred, you can rename the script filename to what you want as long as it’s reflected on the code snippet.
You can also use this defer script in conjunction with other existing scripts with async attributes, so in a perfect implementation you would have two groups of scripts; the first being all the scripts you want executed after page load and the other being scripts that are required for certain core functionalities.
Prioritise Loading Above the Fold Content First
The term “Above the Fold” in web development refers to the first visible section you’re presented with when a webpage loads.
The word “fold” refers to the non-visible sections of a page that require scrolling to get to when a page has loaded.
You can think of the first page of a newspaper as being above the fold since you don’t need to flip through to see the information.
The content above the fold is very important and in most cases is what determines if a reader will continue or stop consuming a piece of content.
You can see this at work in newspaper publishing where all the catchy headlines and stories are presented in big fonts on the first page.
The same is true when a user visits a website, the first few seconds are crucial for them in deciding whether to read on or leave.
Since the content above the fold is the first section they come across, not properly optimizing it can lead to a high bounce rate and poor user experience.
When it comes to loading your site’s content, you should always prioritise the above-the-fold content. Even if your entire page doesn’t load quickly, your user’s will have a better experience if the important information about the page is available to them quickly. This is where techniques like deferring and lazy-loading come in handy, as they work to ensure that the most important elements are rendered first.
Parsing Status: Deferred.
And there you have it, you’ve deferred your parsing! Helpful little trick isn’t it? Now that you’ve got that sorted, you can check your performance metrics to see how big of a speed boost your WordPress site is getting.
If you want to read more tips on web design, user experience, and WordPress, be sure to check out the rest of our blog!
Book a 30-min Introduction Call
Let's jump on a quick intro call We'll break down your project, and pinpoint exactly how we can help.
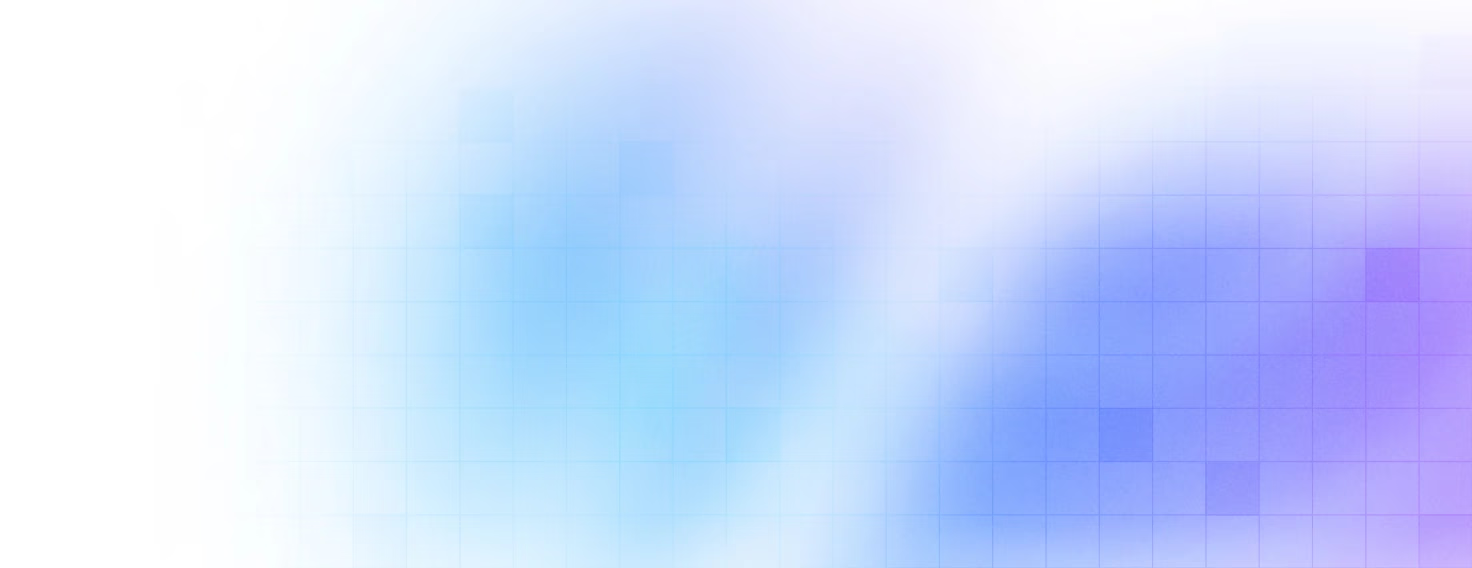
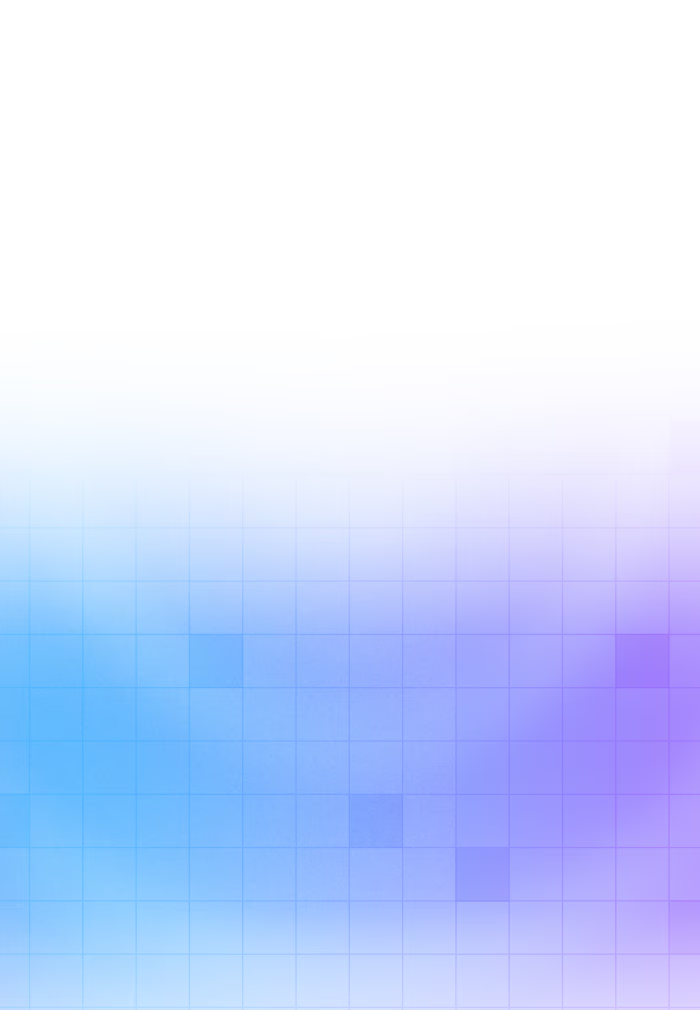
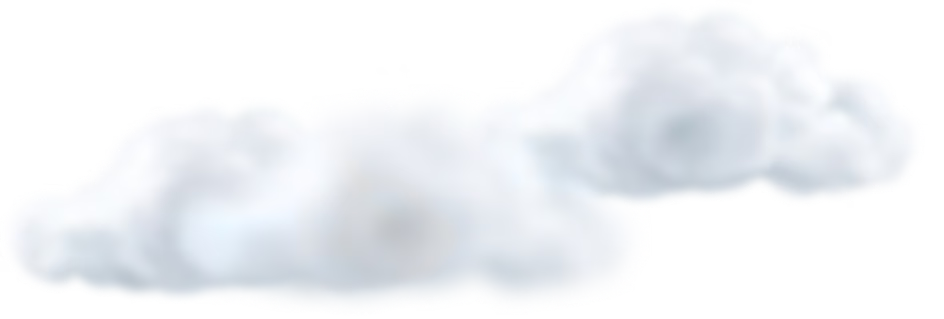
Our clients
Holaa! love working with us see their stories below!
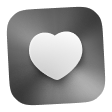
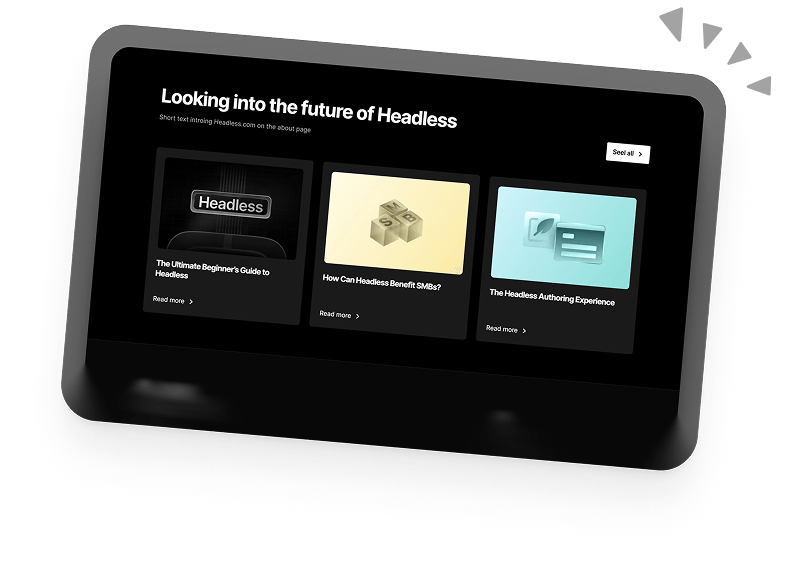